Document Composer, Windows
Sample programs (Windows)
Using the command line
To illustrate the use of the Document Composer, there is a file located in the samples directory that demonstrates how to call it from the command line:
<path-to-mathflow-sdk>/windows/samples/document_composer_cmd32.bat
(for 32-bit systems) <path-to-mathflow-sdk>/windows/samples/document_composer_cmd64.bat
(for 64-bit systems)
About the Script
The document_composer_cmd32.bat (or document_composer_cmd64.bat) file calls the DocumentComposer with several options. It contains the following command (formatted for clarity):
"../bin/DocumentComposer32[or 64]" -license "dessci.lic" -inputdoc "input.html" -outputdoc "output.html" -imagefolder "images" -saveoptions "opts.xml"
The
DocumnentComposer
program is located inbin/DocumentComposer
.The license is a FlexNet license file named
dessci.lic
, located in the same directory as thedocument_composer_cmd32[or 64].bat
file. This option is always required.The
inputdoc
file to be processed is namedinput.html,
also located in the same directory as the.bat
file. This option is always required.The name of the
outputdoc
file will beoutput.html,
also located in the same directory as the.bat
file.Images generated by the MathML in the
inputdoc
file will be written to theimages
subdirectory.Finally, the options used to process this document will be saved to a file named
opts.xml
.
For a complete list of the command line options, see Command line parameters above.
Sample VB.NET application
The sample Visual Basic program below illustrates how to use the DocumentComposer
(DocumentComposer32
or DocumentComposer64
for Windows operating systems) class to process a document with MathML. The source code is provided so that you can experiment with different techniques for use in your own programs.
Prerequisites for running the program
You must have the .NET 2.0 Framework installed.
The
DocumentComposer32.dll
(for Windows 32-bit systems) orDocumentComposer64.dll
(for Windows 64-bit systems) must be registered. Use the commandregsvr32 <path to your operating system's specific DLL>
to register it.The
dessci.lic
file should be in the same directory as the sample code executable. If it's not, be sure to provide the correct path in the License File block of the application window.
Running the program
We don't provide a sample application to illustrate the use of the Document Composer, but since we provide the source code it should be a simple matter to build your own sample application.
To run the program, double-click the file. The sample program allows you to change the input parameters used to process an input document with MathML (see below). After changing the input parameters and telling the program where to save the output document, click the Generate Output Document button.
When the Document Composer successfully processes the document, it will display a confirmation dialog similar to this one:
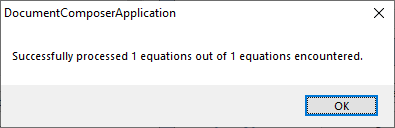
Document Composer tells you how many equations it found and how many it processed in the input document. Dismiss the dialog to see the application window:
<a><img></img></a>Source Code
The source code for the sample program is located in the following directory:
<path-to-mathflow-sdk>/windows/samples/DocumentComposerApplication/src/DocumentComposerApplication
Use Visual Studio to open the DocumentComposerApplication.sln
file.
Programming Notes
The main functions/subroutines in this program are as follows:
New()
Instantiates a
MathFlowSDK.DocumentComposer
object, which is used throughout the program.Initializes the program controls with default values
Sets the options for the Document Composer using the values of the program controls
ProcessDocument()
Within a Try/Catch block:
Sets the options for the Document Composer using the values of the program controls
Calls the method to process the input document
SetOptions()
Sets the value of each option for the Document Composer from the corresponding program control
The general strategy in the sample application for handling exceptions in the
SetOption
methods is:If an option is required (i.e.,
license
orinputdoc
) and an exception is thrown by theSetOption
method, throw an exception with the error message returned from theSetOption
methodIf an option is optional and has a default value (e.g.,
pointsize
), catch any exception thrown by theSetOption
method, retain the warning message for display later and continue processing, using the default value for that optionIf an option is optional and does not have a default value (e.g.,
logfile
), catch any exception thrown by theSetOption
method, retain the resulting message for display later, and call theSetOption
method again with an empty string to tell the system not to use that option and continue processing
Note that this only one way in which the options for a Document Composer can be set. Another possible approach would be to call each set method when the value of a program control changes.
GetOptions()
Sets the value of the program controls from the corresponding options of the Document Composer