Document Composer, Java
Command line parameters (Java)
Command
java DocumentComposer -license <filename | license_key> -inputdoc <filename> [options]
Parameters/Options
Parameter/Option | Description | Default value |
---|---|---|
-license <filename | license_key> | specifies the path and filename for the license file or a license key | required input |
-inputdoc <filename> | specifies the path and file name for the document to be transformed | required input |
-outputdoc <filename> | specifies the path and file name for the transformed document | <inputdoc directory>/inputdoc filename + "_out" + inputdoc suffix |
-outputtype <mathml | images> | specifies whether to write out MathML or image tags | mathml |
-imagefolder <dirpath> | specifies the path to a directory that will contain the image files | <inputdoc directory>/inputdoc filename + "_images"> |
-imagename <filename> | specifies the base file name for the resulting images (e.g. "abc" might result in "abc1.gif") | inputdoc filename + sequence number + "." + imagetype |
-imagetype <gif | png> | specifies the format for the images | gif |
-antialiasingoff <true | false> | Controls whether images will be created with antialiasing or without | false |
-pointsize <int> | specifies the base font point size for the equations | 12 |
-dpi <int> | specifies the resolution of the resulting images in dots per inch | 96 |
-bg <CSS color name | transparent | #rrggbb> | specifies the background color for the equations | white |
-fg <CSS color name | #rrggbb> | specifies the foreground color for the equations | black |
-breakwidth <int> | specifies the width for line wrapping long equations | 0 |
-padding <int> | specifies the padding around the border of the equation images | 0 |
-fontmetrics <true | false> | specifies whether metrics data should be included in the transformed document (it is false when outputtype is set to "images") | true |
-fontmapping <filename> | specifies the path and file name for the font mapping file - If the file does not exist, "FontMapping.opt" will be used instead. If this also does not exist or if a file is invalid, the font associations will remain at their previous values. See Java Rendering Engine for more information. | none |
-charmap <filename> | specifies the full path and file name for a character mapping file | none |
-logfile <filename> | specifies the path and file name for a log file | none |
-verbosity <mute | normal | debug> | specifies what processing messages should be displayed; mute - none; normal - any error messages and whether the image was generated successfully; debug - additional processing information | normal |
-readoptions <filename> | specifies the path and file name of an XML file for reading in options values | none |
-saveoptions <filename> | specifies the path and file name of an XML file for saving out options values | none |
-opdict <filename> | specifies the path and file name of the configurable operator dictionary. See Configuring the operator dictionary for more information. | none |
Sample programs (Java)
Using the command line (Java)
A sample application has been provided to illustrate the use of the Document Composer (for Java), from the command line.
<path-to-mathflow-sdk>/java/samples/document_composer_cmd.bat
(Windows) <path-to-mathflow-sdk>/java/samples/document_composer_cmd.sh
(non-Windows)
About the Script
The Document Composer batch file (or shell script) calls DocumentComposer
with several options. It contains the following command (formatted for clarity):
java -classpath .;../MathFlow.jar com/dessci/mathflow/sdk/composer/DocumentComposer -license dessci.lic -inputdoc input.html -outputdoc output.xhtml
Caution
IMPORTANT NOTES:
This required .jar files needs to be on the
classpath
:MathFlow.jar
(all MathFlow SDK classes)
Within the MathFlow jar file, the
DocumentComposer
program is located atcom/dessci/mathflow/sdk/composer/DocumentComposer
.Your license,
dessci.lic,
is a FlexNet license file that is located in the same directory as the Document Composer batch file (or shell script). This option is always required.The
inputdoc
file to be processed is namedinput.html,
and is located in the same directory as the batch file or shell script. This option is always required.The name of the outputdoc file will be
output.xhtml,
and is located in the same directory as the batch file or shell script.The image generated by the MathML in the
inputdoc
file,input1.gif
, will be written to the defaultinput_images
directory. If this file already exists, it will be overwritten.
For a complete list of the command line options, see Command line parameters.
Sample Java Application
The sample Java program below illustrates how to use the DocumentComposer
class to process a document with MathML. The source code is provided so that you can experiment with different techniques for use in your own programs.
Prerequisites for Running the Program
Java 1.6 or higher
The
dessci.lic
file should be in the same directory as the sample code batch files or shell scripts. If it's not, be sure to enter the correct path in the appropriate spot in the application window.The required .jar file needs to be on the
classpath
:MathFlow.jar
(all MathFlow SDK classes).
Running the Program
<path-to-mathflow-sdk>/java/samples/document_composer_app.bat
(Windows) <path-to-mathflow-sdk>/java/samples/document_composer_app.sh
(non-Windows)
The sample program allows you to change the input parameters used to process an input document with MathML (see below). After changing the input parameters and telling the program where to save the generated images, click the Generate Output Document button.
When the Document Composer successfully processes the document, it will display a confirmation dialog similar to this one:
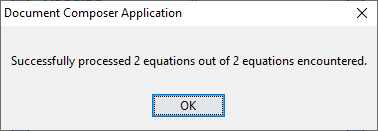
Dismiss the dialog to see the application window:
<a><img></img></a>Source Code
The source code for the sample program is located in:
<path-to-mathflow-sdk>java/samples/com/yourcompany/samplecode/composer/DocumentComposerApplication.java
About the Script
The batch file (or shell script) calls DocumentComposerApplication
.
java -classpath .;../MathFlow.jar
com/yourcompany/samplecode/composer/DocumentComposerApplication
Note the source code for the sample program is located in the following directory:
<path-to-mathflow-sdk>java/samples/com/yourcompany/samplecode/composer/DocumentComposerApplication.java
Programming Notes
The main functions/subroutines in this program are as follows:
processDocument()
This function does the following within a Try/Catch block:
Calls setOptions to set the options for the Document Composer using the values of the program controls
Calls the method to process the input document
setOptions()
This function does the following:
Sets the value of each option for the Document Composer from the corresponding program control
The general strategy in the sample application for handling exceptions in the
SetOption
methods is:If an option is required (i.e.,
license
orinputdoc
) and an exception is thrown by theSetOption
method, throw an exception with the error message returned from theSetOption
methodIf an option is optional and has a default value (e.g.,
pointsize
), catch any exception thrown by theSetOption
method, retain the warning message for display later and continue processing, using the default value for that optionIf an option is optional and does not have a default value (e.g.,
logfile
), catch any exception thrown by theSetOption
method, retain the resulting message for display later and continue processing
Note that this only one way in which the options for a Document Composer can be set. Another possible approach would be to call each set method when the value of a program control changes.
getOptions()
This function does the following:
Sets the value of the program controls from the corresponding options of the Document Composer
readOptions()
This function does the following within a Try/Catch block:
Calls the method to read the options from an XML file
Calls getOptions to set the value of the program controls using the options for the Document Composer
saveOptions()
This function does the following within a Try/Catch block:
Calls setOptions to set the options for the Document Composer using the values of the program controls
Calls the method to save the options to an XML file